Authentication API#
As a standard, if necessary, to identify and authenticate the user, the website or mobile application interacts with Blitz Identity Provider using any of the available protocols (see Selecting an interaction protocol). At the same time, the application does not directly authenticate. The application redirects the user to Blitz Identity Provider to the login page. Next, Blitz Identity Provider independently offers the user various authentication methods, interacts with the user during the login process.
In some cases, it may be desirable to provide the user with the opportunity to complete identification and authentication without being redirected to the Blitz Identity Provider login page. Such capabilities are limited (not all login and login confirmation methods are available without redirection), and require a large amount of improvements on the application side (since the application needs to support the processing of various authentication-related scenarios).
Blitz Identity Provider provides an HTTP API that allows you to embed user identification and authentication into the application’s web page without redirecting the user to a separate login page. This HTTP API is designed for web applications. When using the API, a Web Single Sign‑On is provided, namely, when the user subsequently logs in to another application connected to Blitz Identity Provider in the same web session, he will not be asked to log in again.
Settings for using the API#
The application must be registered in Blitz Identity Provider. The application in Blitz Identity Provider must be assigned client_id
and client_secret
, and the application return URL must be registered in Blitz Identity Provider.
The interaction of the application page and Blitz Identity Provider is based on the execution of a series of AJAX interactions. To enable such interaction, the following CORS (Cross-origin resource sharing
) settings must be made on the application’s web server and on the Blitz Identity Provider web server:
On the Blitz Identity Provider server, for the
/blitz/oauth/ae
handler, you need to configure the CORS permission by adding the following HTTP Headers (you need to specify theorigin
for the PROD site and the necessaryorigin
for the required test environments):"Access-Control-Allow-Origin" -> "https://{app-domain}", "Access-Control-Allow-Credentials" -> "true"
In this header,
{app-domain}
is the application domain.On the portal server, the following CORS permission must be configured for the callback handler (see Interaction scheme) of the response from Blitz Identity Provider (the permission is
null
, since after the redirect the browser resetsorigin
):"Access-Control-Allow-Origin" -> null, "Access-Control-Allow-Credentials" -> "true"
Interaction scheme#
The HTTP authentication API allows you to:
Check for an SSO session. If there is no SSO session, get a list of authentication methods available to the user.
Perform identification and authentication using a username and password.
Perform identification and authentication using a login (phone) and a confirmation code sent by SMS.
Perform identification and authentication using a QR code;
Confirm the login using the confirmation code sent by SMS.
The figure below shows an interaction scheme when logging in with a username and password, followed by confirmation of login using a confirmation code sent via SMS.
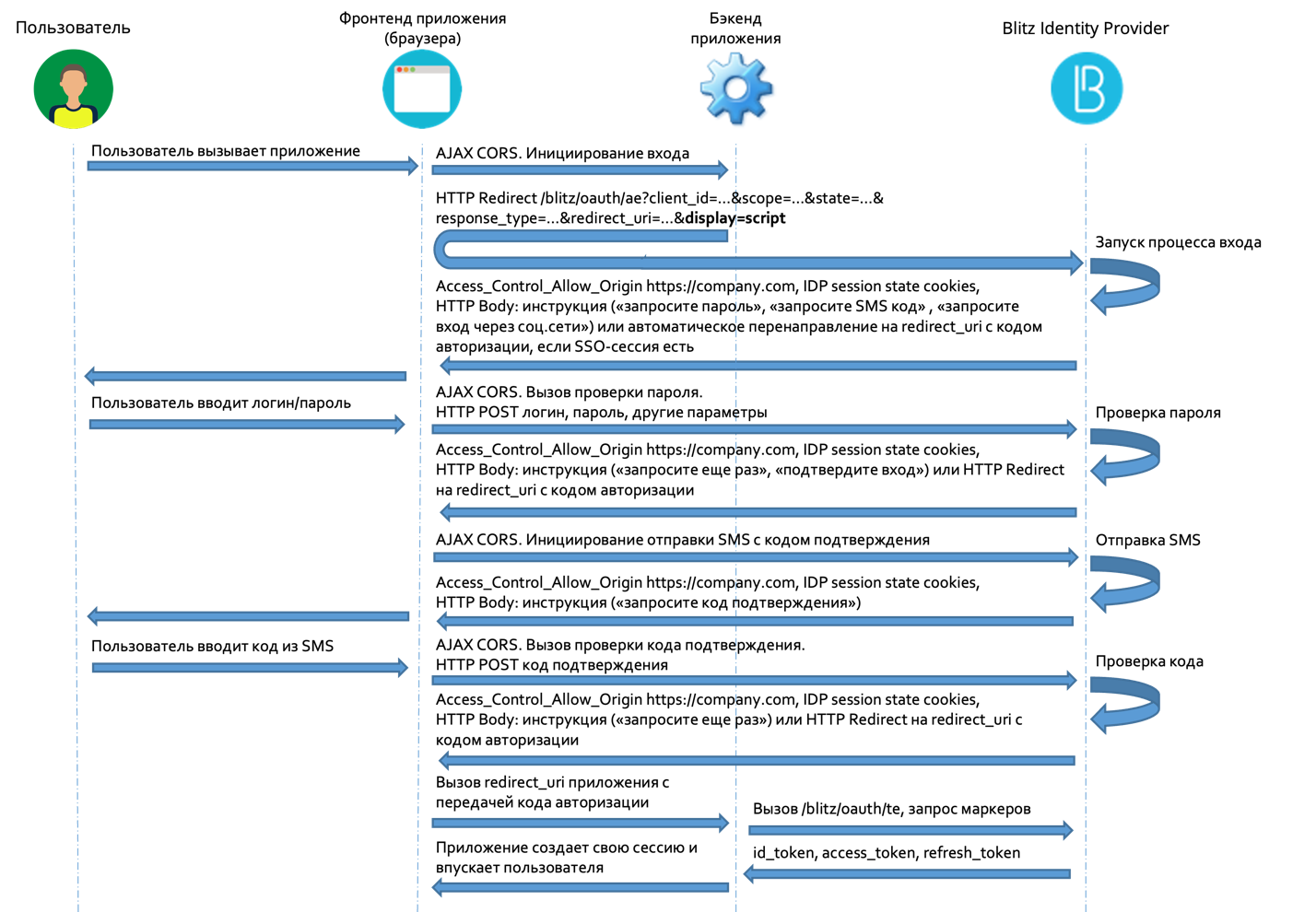
The following figure shows the interaction scheme when logging in by phone and the confirmation code sent by SMS.
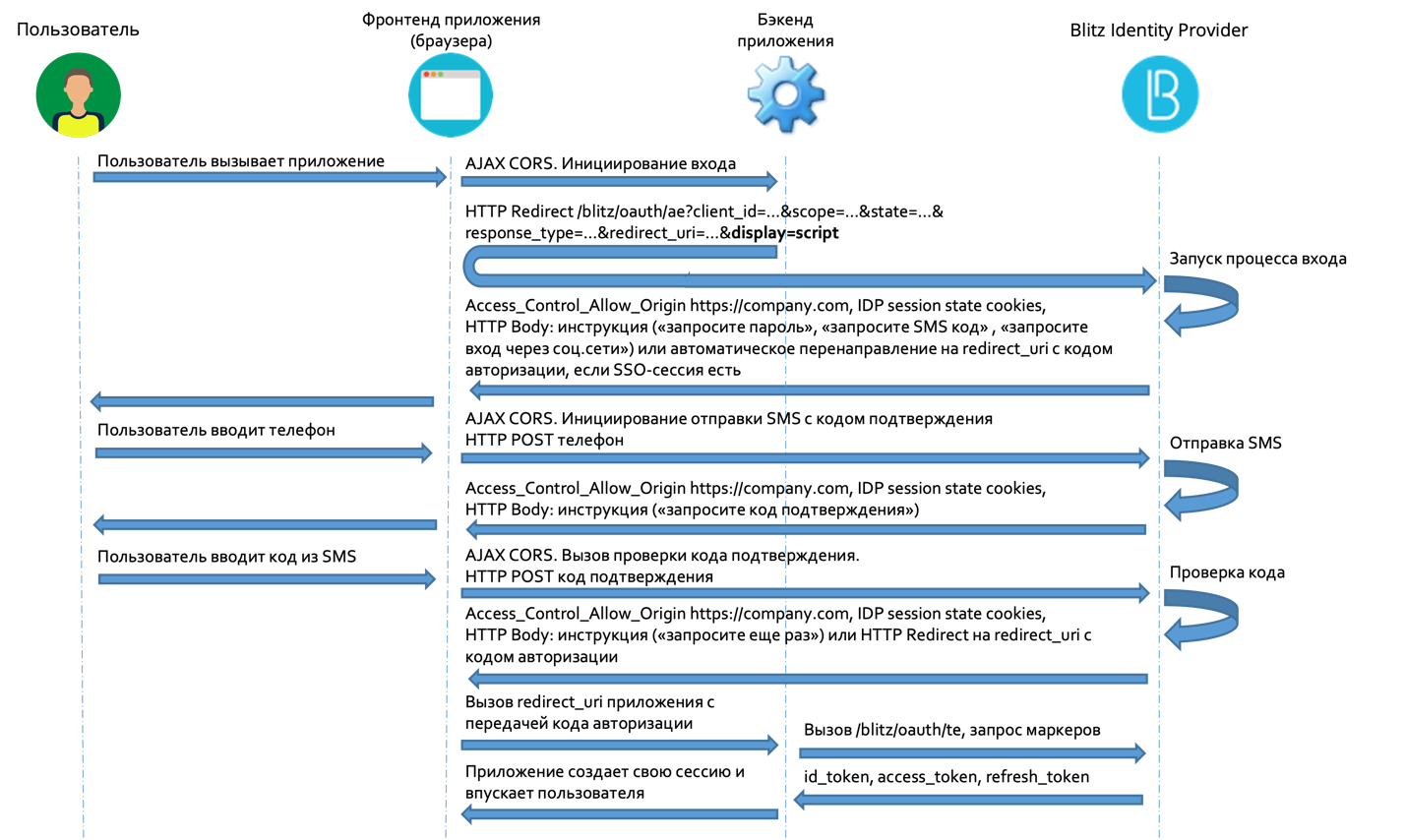
The web application interacts with Blitz Identity Provider by executing a series of AJAX requests.
Note
Requests must be made with the saving and transfer of cookies – you must use withCredentials: true
The following sections describe the requests being called, possible responses, and recommendations for processing them. Examples of requests and responses are provided in the form of cURL calls.
Starting the login process#
To start the login process, the application must send an HTTP GET request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true)
to the usual Authorization Endpoint handler (/blitz/oauth/ae
, see Getting the authorization code), adding the special parameter display=script.
to the request
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request GET 'https://login.company.com/blitz/oauth/ae?response_type=code&client_id=ais&scope=openid&state=…&display=script&redirect_uri=https%3A%2F%2Fapp.company.com%2Fre'
If an SSO session already exists, Blitz Identity Provider will automatically redirect the user to the redirect_uri
handler address, adding the authorization code and the state
parameter to the request. Using the received authorization code, the application will continue the standard OpenID Connect interaction to receive security tokens and account data.
Example of a redirect response if the SSO session already exists:
…
< HTTP/2 302
…
< location: https://…?code=…&state=…
…
An example of a response if authentication is required:
{
"inquire":"choose_one",
"items":[
{
"inquire":"login_with_password"
},
{
"inquire":"request_auth_with_fed_point",
"fp":"esia:esia_1"
},
…
{
"inquire":"request_auth_with_fed_point",
"fp":"yandex:yandex_1"
},
{
"inquire":"login_to_send_sms"
},
{
"inquire":"show_qr_code",
"link":"https://…?code=dde087f0-8f4a-478e-886b-5354b0283362",
"expires":1660905165,
"logo":"https:/…"
}
]
}
If authentication is required, Blitz Identity Provider returns one of the possible instructions to the application:
login_with_password
– log in with your username and password;request_auth_with_fed_point
– log in using an external identification provider (social network);login_to_send_sms
– log in using your username and confirmation code sent via SMS;show_qr_code
– display a QR code that allows you to log in.
If any of the authentication methods are not configured in Blitz Identity Provider or are unavailable for logging into the requesting application (for example, as a result of the settings of the “login procedure” for the corresponding application), then instructions on them will be missing in the service response.
Depending on the security modes included in Blitz Identity Provider, the login_with_password
instruction may contain additional parameters:
If the CAPTCHA mode is configured in Blitz Identity Provider when logging in, then the instructions will contain the
captchaId
parameter that the application needs to use for the CAPTCHA test:
{
"inquire": "choose_one",
"items": [
{
"inquire": "login_with_password",
"captchaId": "9cf48a75-6be1-4008-b34e-8906220c472f"
},
…
]
}
If the password protection mode is configured in Blitz Identity Provider, which requires the application to solve a long-term computational task (Proof of Work), then the
proofOfWork
parameter will be in the instructions:
{
"inquire": "choose_one",
"items": [
{
"inquire": "login_with_password",
"captchaId": "9cf48a75-6be1-4008-b34e-8906220c472f",
"proofOfWork": "1:15:220313184752:abe…539::Ekf…w==:"
}
]
}
If you receive the
proofOfWork
parameter, it is recommended to asynchronously immediately run the algorithm for finding a solution, without waiting for the user to select the login and password login mode and enter the data. This will hide the delay time for solving the problem from the user (it can be several seconds, depending on the complexity of the task). The Hashcash algorithm is currently being used.Important
You need to supplement the proofOfWork parameter with such a value that the hash calculated from it using the SHA-1 algorithm contains at the beginning as many zero bits as specified by the task condition (the number after the first character
:
in theproofOfWork
parameter).For example, the solution for
1:15:yyyy03Su212003:BlitzIdp::McMybZIhxKXu57jd:0
will be the line1:15:yyyy03Su212003:BlitzIdp::McMybZIhxKXu57jd:3/g
Depending on the authentication method selected, the application calls in Blitz Identity Provider login using one of the following methods:
Login via an external identity provider – this type of login is possible only through a browser with redirection of the user to the login page of the external identity provider. You need to repeat the
Authorization Endpoint
call (see Getting the authorization code), use the required value of thebip_action_hint
parameter in the call, corresponding to the external login provider selected by the user (for example,bip_action_hint=externalIdps:esia:esia_1
).
Request example:
https://login.company.com/blitz/oauth/ae?response_type=code&client_id=portal.ru&scope=openid+profile&redirect_uri=https://apitest.company.com/success&state=342a2c0c-d9ef-4cd6-b328-b67d9baf6a7f& bip_action_hint=used_externalIdps:esia:esia_1
In this case, the completion of the login process will occur in a standard way in accordance with OpenID Connect – Blitz Identity Provider will return the authorization code to the redirect_uri
application handler.
Logging in using login and password#
If CAPTCHA is configured in Blitz Identity Provider, then before calling the login and password verification, the application must make calls to receive and verify the CAPTCHA. Verification requests should be generated through specialized proxy services Blitz Identity Provider, and not directly to CAPTCHA services.
When using reCAPTCHA v3, you must initialize reCAPTCHA v3 according to the documentation.
Upload the script on the application page using the same reCAPTCHA v3
sitekey
as registered in Blitz Identity Provider:
<script src="https://www.google.com/recaptcha/api.js?render=reCAPTCHA_site_key"></script>
Call grecaptcha.execute on pressing the login button:
<script>
function onClick(e) {
e.preventDefault();
grecaptcha.ready(function() {
grecaptcha.execute('reCAPTCHA_site_key', {action: 'submit'}).then(function(token) {
// Add your logic to submit to your backend server here.
});
});
}
</script>
Immediately after calling from the reCAPTCHA services login page, you must call the verify operation from the application server. The call should not be made directly to the Google servers, but through a special proxy service in Blitz Identity Provider.
Example of a verification request (operation verify
):
POST /blitz/login/captcha/verify
Content-Type: 'text/json'
{
"ctx": {
// captchaId
"id": "9cf48a75-6be1-4008-b34e-8906220c472f",
"method": "password"
},
"params": {
// token для проверки капчи, полученный при регистрации в Google
"response": "03…sA"
}
}
Ответ ``HTTP 200 OK``:
{
"action": "submit",
"challenge_ts": "2021-03-16T11:18:41Z",
"success": true,
"hostname": "company.com",
"score": 0.9
}
Besides, if Proof of Work protection is enabled in Blitz Identity Provider, then you need to calculate the value of the proofOfWork
parameter (see Starting the login process).
To verify the login and password, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/password
with Content-Type x-www-form-urlencoded
and a Body containing the login
and password
parameters, as well as the calculated proofOfWork
(if this parameter was received from Blitz Identity Provider when starting the login process).
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/password' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'login=логин&password=пароль&proofOfWork=решение'
Blitz Identity Provider performs the necessary security checks upon receipt of the request (whether the CAPTCHA has been passed, whether ProofOfWork
has been resolved, whether the account has been blocked). If the security checks are passed, then Blitz Identity Provider checks the transmitted username and password.
If the login and password checks are successful and if the authentication is sufficient, Blitz Identity Provider will automatically redirect the user to the redirect_uri
handler address, adding the authorization code and the state
parameter to the request. Using the received authorization code, the application will continue the standard OpenID Connect interaction to receive security tokens and account data.
Example of a redirect response if the SSO session already exists:
…
< HTTP/2 302
…
< location: https://…?code=…&state=…
…
If any checks failed or if further actions from the user are required, then Blitz Identity Provider returns one of the instructions.
Example of a response in case of a login and password verification error:
{
"inquire": "login_with_password",
"errors": [
{
"code": "invalid_credentials",
"params": {}
}
]
}
Upon receiving such a response, the application can display the error text and prompt the user to enter another username and password, after which you can repeat the login and password verification.
If the user has entered a password that was previously in the account, or if the account is blocked, the error will look like:
{
"inquire": "login_with_password",
"captchaId": "9cf48a75-6be1-4008-b34e-8906220c472f",
"proofOfWork": "1:15:220313184752:abe…539::Ekf…w==:",
"errors": [
{
"code": "invalid_credentials",
"params": {
"_cause": "used_old_password"
}
}
]
}
An example of getting an error that the CAPTCHA check failed:
{
"inquire": "login_with_password",
"captchaId": "9cf48a75-6be1-4008-b34e-8906220c472f",
"errors": [
{
"code": "invalid_captcha",
"params": {}
}
]
}
An example of an error that the Proof of Work solution was not checked:
{
"inquire": "handle_error",
"errors": [
{
"code": "doesNotMatch",
"params": {}
}
]
}
If special protection is enabled in Blitz Identity Provider to delay the verification of the login and password, then when checking the login and password, you can receive the following instruction from Blitz Identity Provider that you need to re-call the password verification after a certain number of seconds:
{
"inquire": "delayed_login_with_password",
"delayedFor": 5
}
A repeat call must be made when the required time has passed. The isDelayed=true
parameter must be passed to the repeated call.
Example of calling password verification again in response to the instruction delayed_login_with_password:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/password' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'login=логин&password=пароль&proofOfWork=решение&isDelayed=true'
If special password brute force protection is enabled in Blitz Identity Provider, then Blitz Identity Provider may request additional CAPTCHA verification when verifying the password for this account. There are two possible situations:
The user passed the wrong password, after which the protection turned on, and the CAPTCHA is needed for another authentication attempt.
User account password brute force protection was enabled earlier. The current transmitted password was not verified because the CAPTCHA test was not performed.
In the first case, you need to inform the user that the username and password are incorrect, and for a new attempt, in addition to entering the password, request to take a CAPTCHA test.
In the second case, you need to ask the user to take a CAPTCHA test, and then send the previously entered username and password for verification.
An example of the answer for the first case is that the password is incorrect and a CAPTCHA test is needed:
{
"inquire":"login_with_password",
"captchaId":"1c9e4047-c8c4-47ad-a447-cc1809bd3e6c",
"errors":[
{
"code":"invalid_credentials",
"params":{}
}
]
}
An example of the answer for the second case is that the password was not checked and a CAPTCHA test is needed:
{
"inquire":"login_with_password",
"captchaId":"2f818f5d-3a89-428d-b424-cde38c19051e",
"errors":[
{
"code":"bypass_captcha",
"params":{}
}
]
}
An example of an error if the account is temporarily blocked:
{
"inquire":"login_with_password",
"errors": [
{
"code":"pswd_method_temp_locked",
"params":{"0":"2"}
}
]
}
An example of an error if the account is blocked due to prolonged inactivity:
{
"inquire": "handle_error",
"errors": [
{
"code": "inactivity_lock",
"params": {}
}
]
}
If the account password does not match the password policy, it may be necessary to change the password when logging in. In this case, Blitz Identity Provider will return instructions that you need to redirect the user to the page with the specified address:
{
"inquire":"go_to_web",
"redirect_uri":
"https://…/blitz/login/methods/password/change?f=false&c=password_policy_violated"
}
If the login and password are successful, but you additionally need to confirm the login, then instructions will be returned with possible confirmation methods:
{
"inquire":"choose_one",
"items": [
{
"inquire":"ask_to_send_sms"
},
{
"inquire":"go_to_web",
"redirect_uri":"https://login.company.com/blitz/login/methods2/sms"
}
]
}
You can either redirect the user to a web page so that he continues to confirm login on the Blitz Identity Provider web page, or continue to use the HTTP API to confirm login by SMS code.
If the login procedure set for the application is configured to invoke an additional screen after logging in (for example, see Invoking the auxiliary application at the moment of login). Invoking the auxiliary application at the time of login), then Blitz Identity Provider redirects the user to this screen.
Login by phone and confirmation code#
Logging in by phone and confirmation code consists of the following steps:
Sending a confirmation code to the user via SMS.
Verification of the confirmation code entered by the user.
To send the user a confirmation code via SMS, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/sms/bind
with Content-Type x-www-form-urlencoded
and a Body containing the user’s login
. It is recommended to pass the phone number entered by the user as the login
.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'login=логин'
If the account with the transmitted username is not found, then Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"no_subject_found",
"params":{}
}
]
}
If the account is found, but a search of confirmation codes was previously recorded for it, then Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"method_temp_locked",
"params":{}
}
]
}
If the account is found and it is possible to log in this way, then Blitz Identity Provider will send the user an SMS with a confirmation code and return a response:
{
"inquire":"enter_sms_code",
"contact":"+79991234567",
"ttl":300,
"remain_attempts":3
}
The received response indicates how many seconds the user has left to send the code for verification (ttl), how many attempts he has to enter the code (remain_attempts), to which phone number the code was sent to him (contact
).
To verify the confirmation code entered by the user, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/sms/bind
with Content-Type x-www-form-urlencoded
and a Body containing an sms-code
with a confirmation code.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'sms-code=123456'
If the code is incorrect, then Blitz Identity Provider will return an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"invalid_otp",
"params":{}
}
],
"contact":"+79991234567",
"remain_attempts":2,
"ttl":276
}
If the number of code verification attempts has ended, Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"no_attempts",
"params":{}
}
]
}
If the code has expired, Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"expired",
"params":{}
}
]
}
In case of this error, you can request to send a new confirmation code. To do this, the application must call Blitz Identity Provider as follows:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'sms-send=sms'
If you request to resend the code before the expiration of the previous one, an error will be returned:
{
"inquire":"handle_error",
"errors":[
{
"code":"code_not_expired",
"params":{}
}
]
}
If the total number of attempts to log in using the confirmation code from the SMS is exceeded, then Blitz Identity Provider temporarily blocks the login for the account using the confirmation code. In this case, the next time you try to enter an incorrect confirmation code, Blitz Identity Provider may return an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"method_temp_locked",
"params":{}
}
]
}
If the entered confirmation code is correct, and this is enough to complete the login, then Blitz Identity Provider will automatically redirect the user to the redirect_uri
handler address, adding the authorization code and the state
parameter to the request. Using the received authorization code, the application will continue the standard OpenID Connect interaction to receive security tokens and account data.
Example of a response in case of a successful login:
…
< HTTP/2 302
…
< location: https://…?code=…&state=…
…
If the verification of the confirmation code is successful, but you additionally need to confirm the login, an instruction will be returned with possible confirmation methods:
{
"inquire":"choose_one",
"items":[
{
"inquire":"go_to_web",
"redirect_uri":"https://login.company.com/blitz/login/methods2/email"
}
]
}
Logging in with email#
Logging in using email consists of the following steps:
Emailing a confirmation code to a user .
Verification of the confirmation code entered by the user.
To send the user a confirmation code via email, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/email/bind
with Content-Type x-www-form-urlencoded
and a Body containing the user’s login
. It is recommended to pass the email entered by the user as the login
.
Request example:
curl --location --request POST 'https://login.company.com/blitz/login/methods/headless/email/bind' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'login=<email>'
Response example:
{
"inquire": "enter_email_code",
"contact": "user@gmail.com",
"remain_attempts": 3,
"ttl": 300
}
Code verification:
curl --location --request POST 'https://login.company.com/blitz/login/methods/headless/email/bind' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'email-code=746234'
Response options if the verification failed:
{
"errors": [
{
"code": "invalid_otp",
"params": {}
}
],
"contact": "user@gmail.com",
"inquire": "handle_error",
"remain_attempts": 2,
"ttl": 257
}
{
"inquire": "handle_error",
"errors": [
{
"code": "no_attempts",
"params": {}
}
]
}
Resubmitting the code:
curl --location --request POST 'https://login.company.com/blitz/login/methods/headless/email/bind' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--header 'Cookie: blc=Hc..; bua=7cd2c312-...; cTm=1:RGVm==; cTmTgs=1:c3Nv; oauth_az=0MyeV-5v_...OnIE; portal_lang=ru' \
--data-urlencode 'email-send=email'
Response to the code resubmission:
{
"inquire": "enter_email_code",
"contact": "user@gmail.com",
"remain_attempts": 1,
"ttl": 288
}
Login by QR code#
Login by QR code consists of the following steps:
Displaying a QR code to the user on the computer where the login is performed;
Periodic check whether the user has scanned the QR code with the mobile application;
Periodic verification of whether the user has confirmed or rejected the QR code login request in the mobile application;
Updating an outdated QR code.
The application should display the QR code to the user by encoding the string received from Blitz Identity Provider into it. Below is a fragment of the instructions for logging in using a QR code (see Starting the login process).
{
"inquire":"choose_one",
"items":[
…
{
"inquire":"show_qr_code",
"link":"https://…?code=dde087f0-8f4a-478e-886b-5354b0283362",
"expires":1660905165,
"logo":"https:/…"
}
]
}
Explanations of the parameters received from Blitz Identity Provider:
inquire
is an instruction with an available login option, in case of login using a QR code, the value isshow_qr_code
;link
is a link that should be encoded in a QR code displayed to the user;expires
is the time (in Unix Epoch) until which the QR code is valid. After the expiration date, it is recommended to display to the user that the QR code is expired;logo
– if Blitz Identity Provider is configured to display a small logo in the center on top of the QR code, then the URL of the logo will be returned in the specified setting.
When the application displays the QR code to the user, it is necessary to wait for the user to read the QR code with a special mobile application. The integration of the mobile application for embedding the QR code login function is described in Login to the application using a QR code.
The web application can periodically check whether the QR code has been read by the mobile application. To do this, you need to execute an HTTP GET request in AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/qrCode/pull
.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request GET 'https://login.company.com/blitz/login/methods/headless/qrCode/pull'
If the QR code has not been read yet, the response will be returned:
{
"command":"showQRCode"
}
If the QR code is read, the response will be returned:
{
"command":"askForConfirm"
}
In this case, you can update the user’s web page and write on it that confirmation of login in the mobile application is expected.
If the QR code is expired, the response will be returned:
{
"command":"needRefresh",
"cause":"qr_code_expired"
}
If the user rejected the QR code login request in the mobile application, the response will be returned:
{
"command":"needRefresh",
"cause":"refused_login"
}
If the QR code is expired or the user declined to log in using the QR code, then you can ask the user to get a new QR code. To do this, run an HTTP POST request in AJAX to Blitz Identity Provider (required with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/qrCode/refresh
.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/qrCode/refresh'
Response example:
{
"link":"https://…?code=4ddf1667-d57f-4f86-b8f2-3ee53b367dfe",
"expires":1660922807,
"logo":"https:/…"
}
If the user has confirmed the QR code login request in the mobile application, then the service https://login.company.com/blitz/login/methods/headless/qrCode/pull
will return the response:
{
"command":"needComplete"
}
In response to this request, to complete the login, an HTTP POST request must be executed in AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/qrCode/complete
.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/qrCode/complete'
If the authentication is sufficient to complete the login, Blitz Identity Provider will automatically redirect the user to the redirect_uri
handler address, adding the authorization code and the state
parameter to the request. Using the received authorization code, the application will continue the standard OpenID Connect interaction to receive security tokens and account data.
Example of a response in case of a successful login:
…
< HTTP/2 302
…
< location: https://…?code=…&state=…
…
If you need to go through additional login confirmation, instructions will be returned with possible confirmation methods:
{
"inquire":"choose_one",
"items":[
{
"inquire":"go_to_web",
"redirect_uri":"https://login.company.com/blitz/login/methods2/email"
}
]
}
Confirmation of login by confirmation code#
Confirmation of the login using the SMS confirmation code consists of the following steps:
Sending a confirmation code to the user via SMS.
Verification of the confirmation code entered by the user.
To send the user a confirmation code via SMS, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/sms/bind
with Content-Type x-www-form-urlencoded
without Body:
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded"
Blitz Identity Provider will send the user an SMS with a confirmation code and return a response:
{
"inquire":"enter_sms_code",
"contact":"+79991234567",
"ttl":300,
"remain_attempts":3
}
The received response indicates how many seconds the user has left to send the code for verification (ttl), how many attempts he has to enter the code (remain_attempts), to which phone number the code was sent to him (contact
).
To verify the confirmation code entered by the user, the application must send an HTTP POST request to AJAX to Blitz Identity Provider (necessarily with withCredentials: true
) on the URL https://login.company.com/blitz/login/methods/headless/sms/bind
with Content-Type x-www-form-urlencoded
and a Body containing an sms-code
with a confirmation code.
Request example:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'sms-code=123456'
If the code is incorrect, then Blitz Identity Provider will return an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"invalid_otp",
"params":{}
}
],
"contact":"+79991234567",
"remain_attempts":2,
"ttl":276
}
If the number of code verification attempts has ended, Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"no_attempts",
"params":{}
}
]
}
If the code has expired, Blitz Identity Provider returns an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"expired",
"params":{}
}
]
}
In case of this error, you can request to send a new confirmation code. To do this, the application must call Blitz Identity Provider as follows:
curl -v -b cookies.txt -c cookies.txt \
--request POST 'https://login.company.com/blitz/login/methods/headless/sms/bind' \
--header "Content-Type: application/x-www-form-urlencoded" \
--data 'sms-send=sms'
If you request to resend the code before the expiration of the previous one, an error will be returned:
{
"inquire":"handle_error",
"errors":[
{
"code":"code_not_expired",
"params":{}
}
]
}
If the total number of attempts to confirm login by SMS confirmation code is exceeded, then Blitz Identity Provider temporarily blocks login confirmation for the account by confirmation code. In this case, the next time you try to enter an incorrect confirmation code, Blitz Identity Provider may return an error:
{
"inquire":"handle_error",
"errors":[
{
"code":"method_temp_locked",
"params":{}
}
]
}
If the entered confirmation code is correct, and this is enough to complete the login, then Blitz Identity Provider will automatically redirect the user to the redirect_uri
handler address, adding the authorization code and the state
parameter to the request. Using the received authorization code, the application will continue the standard OpenID Connect interaction to receive security tokens and account data.
Example of a response in case of a successful login:
…
< HTTP/2 302
…
< location: https://…?code=…&state=…
…